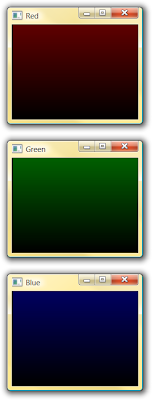
The code hasn't been edited much. Just added one for loop to draw scan lines of the same color (that gives another idea). However, the coordinates are set to 200 x 150 where as the window size is set to 400 x 300. This makes the code to draw like this:
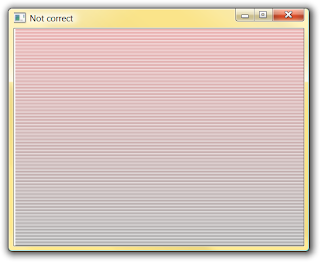
This is not correct. If you click on the image, it will show it full size, where every other line is left totally blank. So, I edited the window size to be 200 x 150 too. Although, I could have changed the coordinates range too.
Another note, for this wrong example, I edited the background to white, like the previous code, instead of keeping it black in the correct versions.
Here's the code:
#include <GL/glut.h> void init (void) { glClearColor (1.0, 1.0 ,1.0, 0.0); glMatrixMode (GL_PROJECTION); gluOrtho2D (0., 200.0, 0.0, 150.0); // the coordinate range } void lineSegment (void) { int count; float col = 0.0; glClear (GL_COLOR_BUFFER_BIT); glBegin (GL_LINES); for (count = 0; count < 201; count += 1){ glColor3f (col, 0.0, 0.0); glVertex2i (1, count); glVertex2i (200, count); col = col + 0.005; } glEnd(); glFlush(); } void main (int argc, char** argv) { glutInit(&argc, argv); glutInitDisplayMode (GLUT_SINGLE | GLUT_RGB); glutInitWindowPosition (50, 100); // top-left window position glutInitWindowSize(200, 150); // window size glutCreateWindow ("Red"); init(); glutDisplayFunc (lineSegment); glutMainLoop (); }
0 comments:
Post a Comment